DatePicker
A DatePicker is a control that allows you to select a date. In the following, MinimumDate is the lowest selectable date for the DatePicker, which defaults to the first day of the year 1900. MaximumDate is the highest selectable date for the DatePicker, which defaults to the last day of the year 2100. Date is the selected date, which defaults to the value DateTime.Today. DateSelected is the event to fire when a date is selected.
<DatePicker MinimumDate="01/01/2020"
MaximumDate="12/31/2025"
Date="02/10/2022"
DateSelected="OnDateSelected" />
When specifying a DateTime in XAML, a InvariantCulture:Date="02/10/2022" (two-digit months, two-digit days, and four-digit years) is assumed
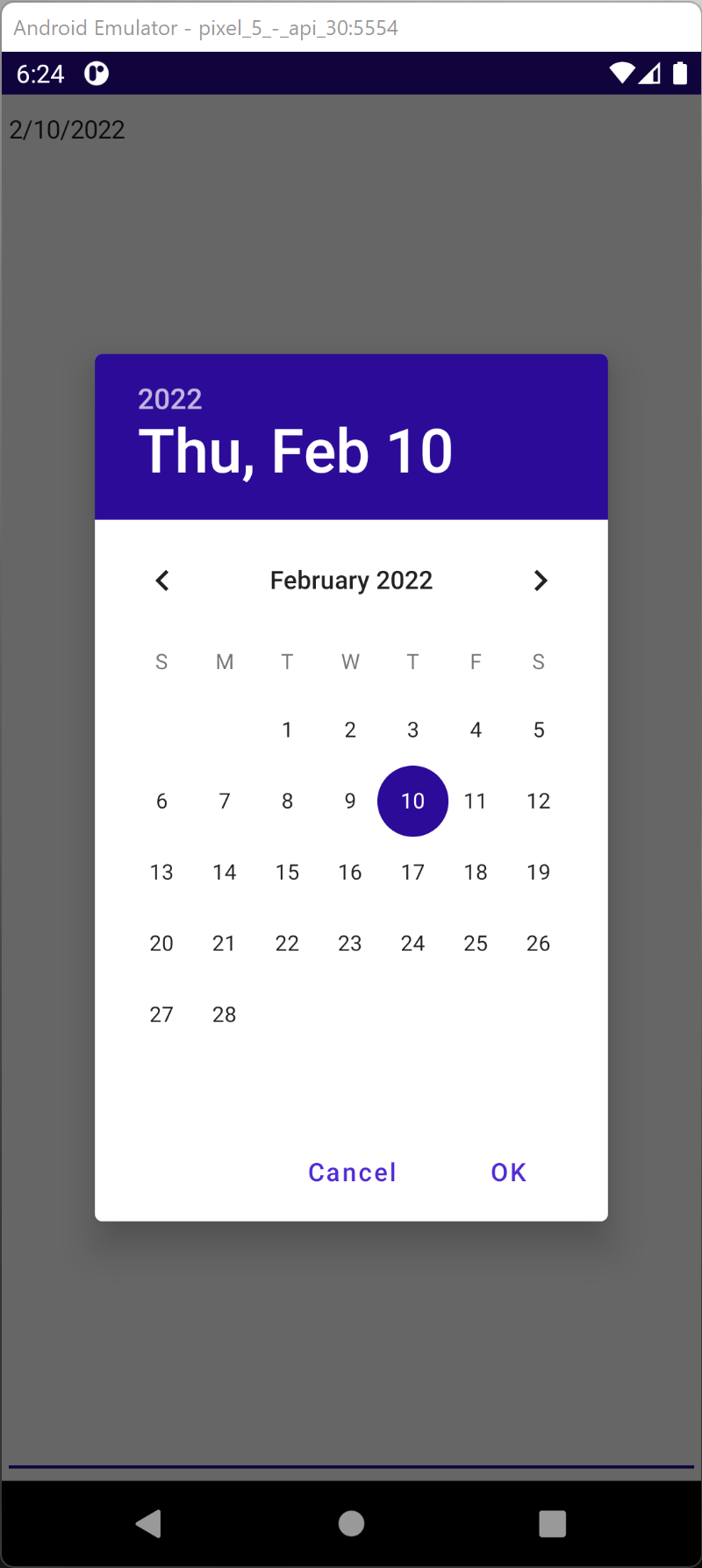
void OnDateSelected(object sender, DateChangedEventArgs args)
{
Console.WriteLine(((DatePicker)sender).Date);
Console.WriteLine(((DatePicker)sender).Date.ToString("d"));
Console.WriteLine(((DatePicker)sender).Date.ToString("D"));
Console.WriteLine(((DatePicker)sender).Date.ToString("f"));
Console.WriteLine(((DatePicker)sender).Date.ToString("F"));
CultureInfo culture = new CultureInfo("en-SG");
Console.WriteLine(((DatePicker)sender).Date.ToString("d", culture));
culture = CultureInfo.InvariantCulture;
Console.WriteLine(((DatePicker)sender).Date.ToString("d", culture));
}
A standard date and time format string using a single character as the format specifier is used above to define the text representation of the date. The results are shown below:
[DOTNET] 2/23/2022 12:00:00 AM
[DOTNET] 2/23/2022
[DOTNET] Wednesday, February 23, 2022
[DOTNET] Wednesday, February 23, 2022 12:00 AM
[DOTNET] Wednesday, February 23, 2022 12:00:00 AM
[DOTNET] 23/2/2022
[DOTNET] 02/23/2022
Date Formatting
- d - Short Date Pattern e.g. 2/23/2022
- D - Long Date Pattern e.g. Wednesday, February 23, 2022
- f - Full Date/Short Time Pattern e.g. Wednesday, February 23, 2022 12:00 AM
- F - Full Date/Long Time Pattern e.g. Wednesday, February 23, 2022 12:00 AM
Date CultureInfo
In the following, the Singapore CultureInfo and InvariantCulture is used for formatting the date.
void OnDateSelected(object sender, DateChangedEventArgs args)
{
.
.
.
CultureInfo culture = new CultureInfo("en-SG");
Console.WriteLine(((DatePicker)sender).Date.ToString("d", culture));
culture = CultureInfo.InvariantCulture;
Console.WriteLine(((DatePicker)sender).Date.ToString("d", culture));
}
Download