Entry Handler
.NET MAUI controls are rendered via handlers, which are lightweight classes that map appearance, properties, and behavior to native implementations. Through these handlers, you can change the look and feel of the native platform control easily. The sample below illustrates how to create an Entry Handler and then used it to change its background color.
1. In your .NET MAUI Project, create a MyEntry class that inherits from Entry.
namespace entryhandler
{
public class MyEntry: Entry
{
}
}
2. In MainPage.xaml, add a MyEntry element for entering text. An entry namespace is declared with the following:
xmlns:entry="clr-namespace:entryhandler"
With the namespace, MyEntry can be use in XAML as shown inside the StackLayout below:
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="entryhandler.MainPage"
xmlns:entry="clr-namespace:entryhandler"
BackgroundColor="{DynamicResource SecondaryColor}">
<StackLayout x:Name="MyStackLayout"
Margin="10">
<Label Text="Name" />
<entry:MyEntry
Text="MauiMan Entry Handler"/>
</StackLayout>
</ContentPage>
3. We have defined an Entry subclass and use it in a XAML page. The aim is so that we can change the appearance of the Entry control. In App.cs, add the following:
using Microsoft.Maui.Platform;
namespace entryhandler;
public partial class App : Application
{
public App()
{
InitializeComponent();
Microsoft.Maui.Handlers.EntryHandler.EntryMapper.AppendToMapping(nameof(IView.Background), (handler, view) =>
{
if (view is MyEntry)
{
#if ANDROID
handler.NativeView.SetBackgroundColor(Colors.LightGray.ToNative());
#elif IOS
handler.NativeView.BackgroundColor = Colors.LightGray.ToNative();
handler.NativeView.BorderStyle = UIKit.UITextBorderStyle.Line;
#elif WINDOWS
handler.NativeView.Background = Colors.LightGray.ToNative();
#endif
}
});
MainPage = new MainPage();
}
}
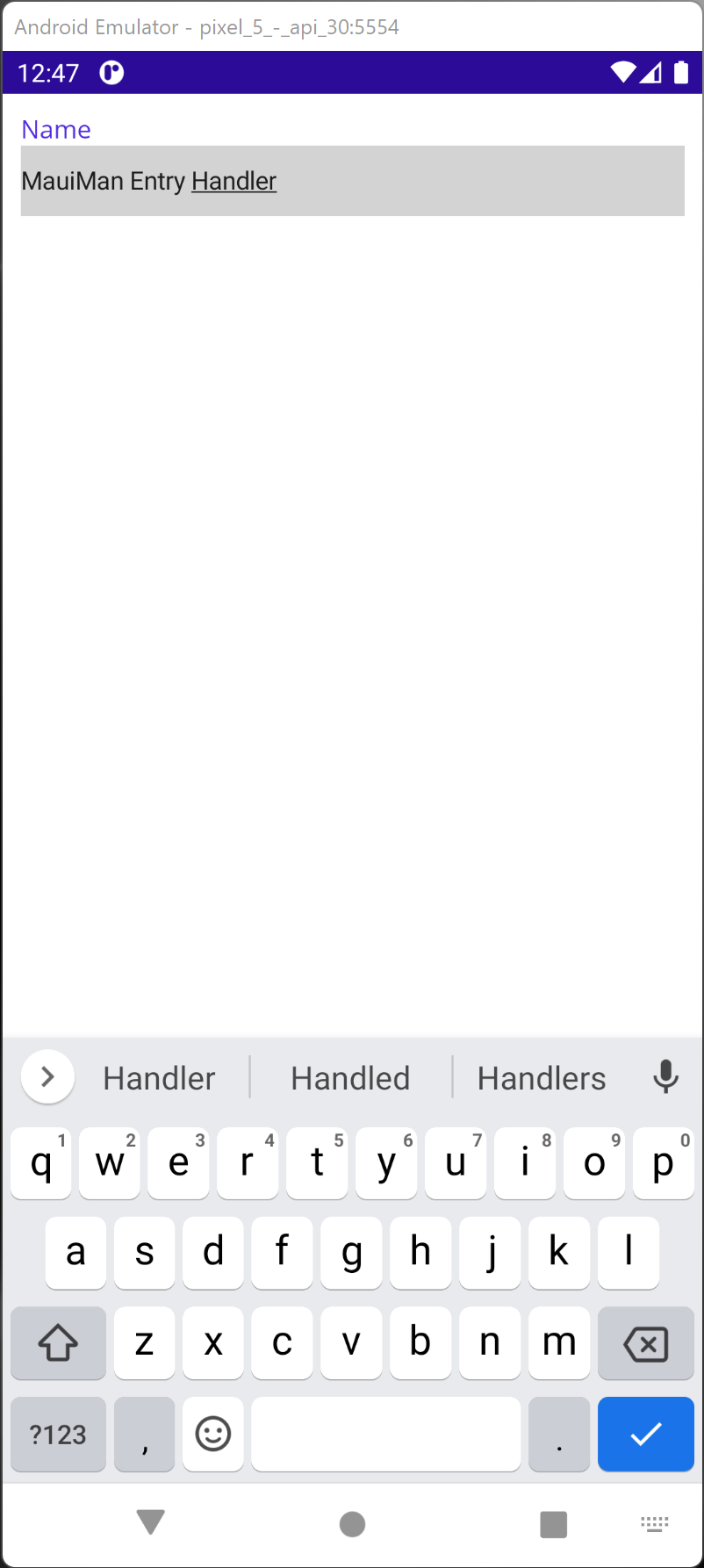
4. (Optional: Platform-Specific) You can also consider using platform-specific code for changing the appearance of a control. For example, you can modify MainActivity.cs with the following Entry Handler code:
using Microsoft.Maui;
using Microsoft.Maui.Platform;
.
.
public class MainActivity : MauiAppCompatActivity
{
public MainActivity()
{
Microsoft.Maui.Handlers.EntryHandler.EntryMapper.AppendToMapping(nameof(IView.Background), (handler, view) =>
{
if (view is MyEntry)
{
handler.NativeView.SetBackgroundColor(Colors.LightGray.ToNative());
}
});
}
.
.
}
Download