Picker
A Picker is a control for selecting a text item from a list of data.
<StackLayout>
<Picker
x:Name="FruitsPicker"
Title="Select a Fruit"
TitleColor="Blue"
ItemDisplayBinding="{Binding FruitName}"
SelectedItem="{Binding SelectedFruit}"
SelectedIndexChanged="OnSelectedIndexChanged" />
</StackLayout>
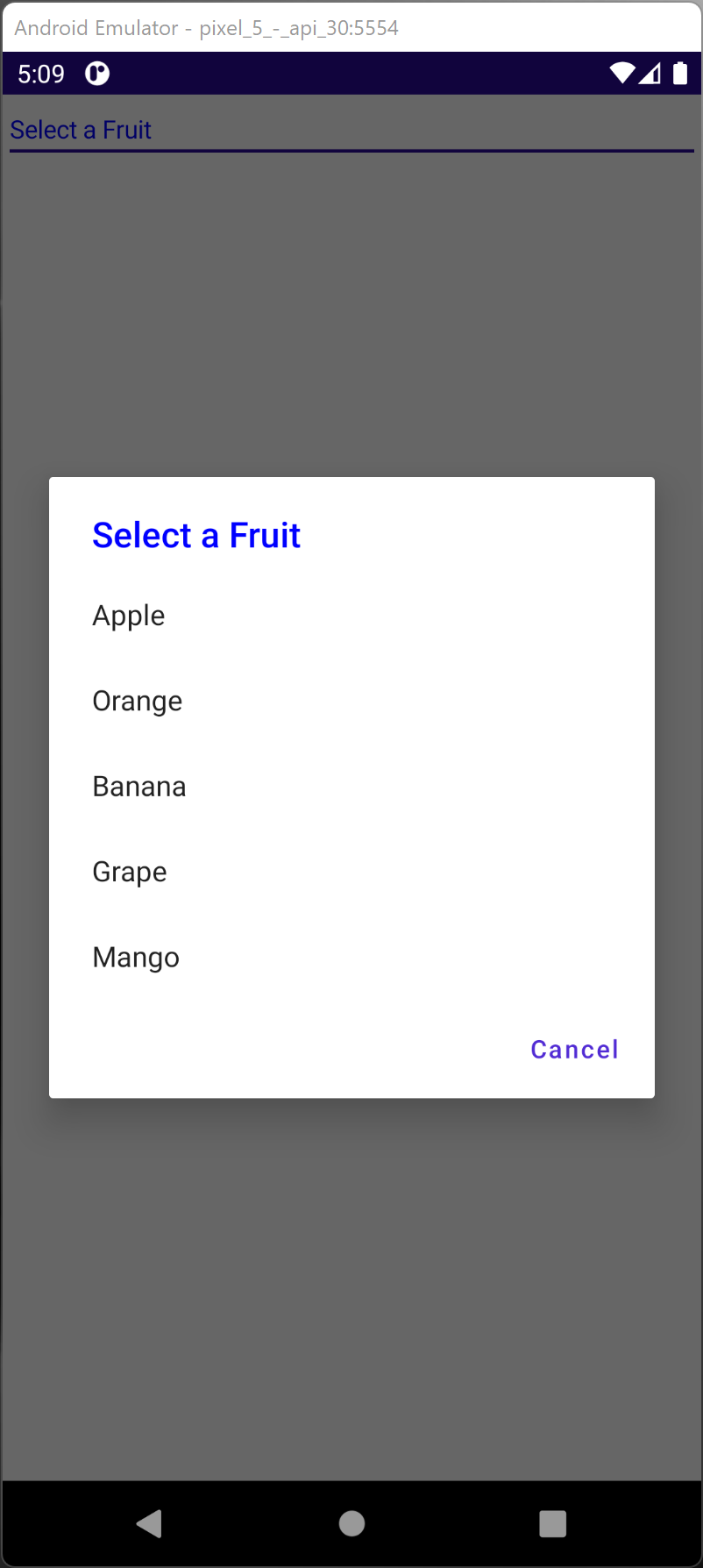
The ItemDisplayBinding property allows the Picker to display text for the objects it is bounded to. The Picker fires a SelectedIndexChanged event when the user selects an item. The SelectedItem property enables you to get or set the selected item from the list of data.
Code Behind
public partial class MainPage : ContentPage
{
public class Fruit
{
public string FruitName { get; set; }
public string FruitDescription { get; set; }
}
ObservableCollection fruits = new ObservableCollection();
public ObservableCollection Fruits { get { return fruits; } }
public MainPage()
{
InitializeComponent();
fruits.Add(new Fruit() { FruitName = "Apple", FruitDescription = "An apple is an edible fruit produced by an apple tree (Malus domestica)." });
fruits.Add(new Fruit() { FruitName = "Orange", FruitDescription = "The orange is the fruit of various citrus species in the family Rutaceae." });
fruits.Add(new Fruit() { FruitName = "Banana", FruitDescription = "A long curved fruit with soft pulpy flesh and yellow skin when ripe." });
fruits.Add(new Fruit() { FruitName = "Grape", FruitDescription = "A berry growing in clusters on a grapevine, eaten as fruit and used in making wine." });
fruits.Add(new Fruit() { FruitName = "Mango", FruitDescription = "A mango is an edible stone fruit produced by the tropical tree Mangifera indica." });
FruitsPicker.ItemsSource = fruits;
}
private void OnSelectedIndexChanged(object sender, EventArgs e)
{
Fruit selectedFruit = ((Picker)sender).SelectedItem as Fruit;
Console.WriteLine(selectedFruit.FruitName);
}
}
The picker is populated with data by setting the ItemsSource property.
Download