XAML Style
When we style the controls in the different pages of our MAUI app, we often need to repeat a lot of code to achieve styling consistency. For example, if we need to make our title use a Large FontSize and Bold FontAttributes, we need to repeat the following code in each page of our app.
<Label Text="My Page Title"
FontSize="Large"
FontAttributes="Bold" />
Alternatively, we can define a global styling in App.xaml:
<Style x:Key="TitleLabelStyle" TargetType="Label">
<Setter Property="FontSize" Value="Large"></Setter>
<Setter Property="FontAttributes" Value="Bold"></Setter>
</Style>
And then in each of the page such as MainPage.xaml, we can use the TitleLabelStyle with a StaticResource keyword. This will result in significant reduction of XAML code especially in situations where we have a lot of styling.
<Label Text="Title"
Style="{StaticResource TitleLabelStyle}" />
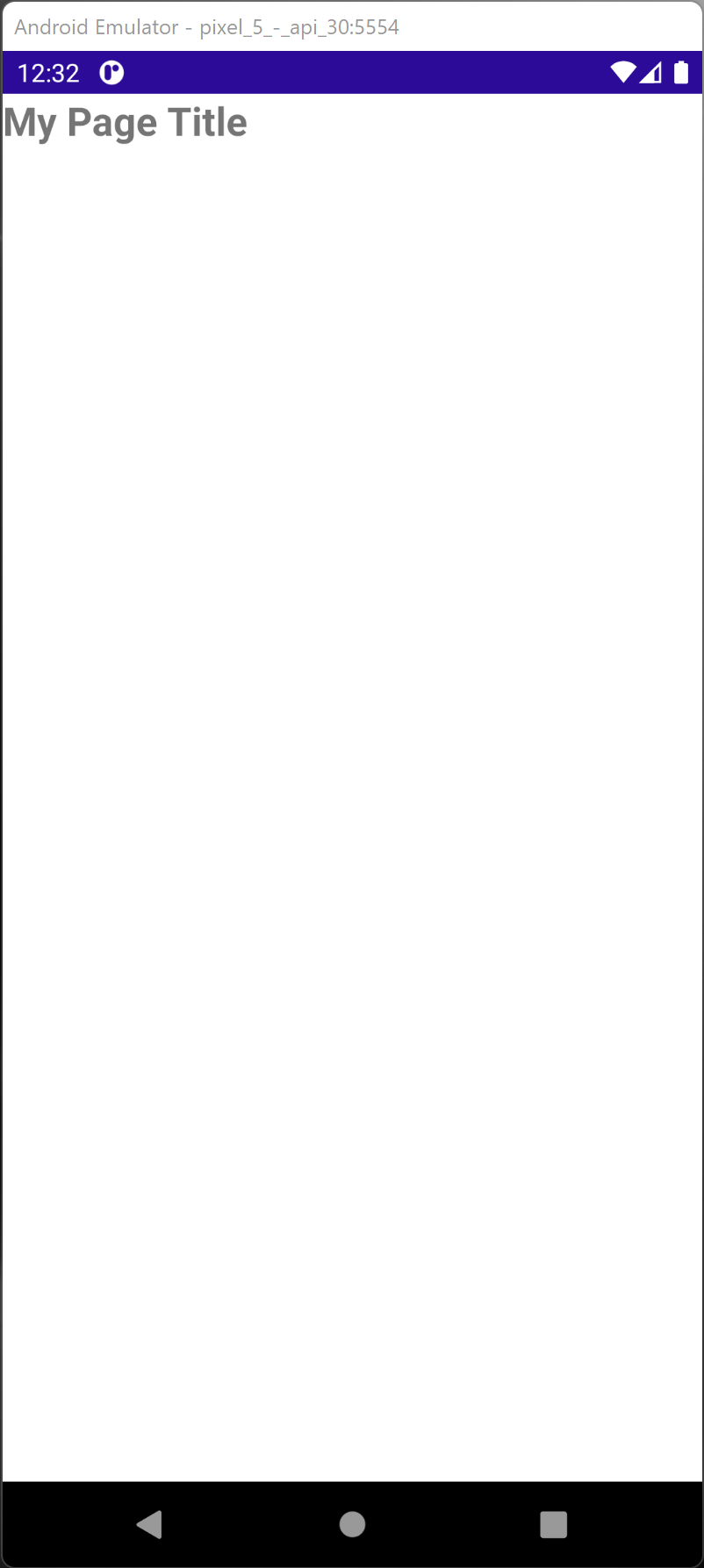
Implicit Style
In the above, the style is optional and we can choose whether to use it with the StaticResource keyword. Another type of style available is the implicit style. This type of style applies to the type of control without you needing to specify the StaticResource keyword. The Implicit Style is shown below in App.xaml (note the lack of x:Key).
<Style TargetType="Label">
<Setter Property="FontSize" Value="Large"></Setter>
<Setter Property="FontAttributes" Value="Bold"></Setter>
</Style>
Now when you specify a title, you will only need the following:
<Label Text="Title" />
XAML Style Inheritance
A XAML style can also serves as a base style for other styles. For example, we can define a RedTitleLabelStyle that inherits all the properties of a BaseTitleLabelStyle.
<Style x:Key="BaseTitleLabelStyle" TargetType="Label">
<Setter Property="FontSize" Value="Large"></Setter>
<Setter Property="FontAttributes" Value="Bold"></Setter>
</Style>
<Style x:Key="RedTitleLabelStyle" TargetType="Label"
BasedOn="{StaticResource BaseTitleLabelStyle}">
<Setter Property="TextColor" Value="Red" />
</Style>
When you use the following in MainPage.xaml, the Label will be Large, Bold and Red.
<Label Text="Title"
Style="{StaticResource RedTitleLabelStyle}" />
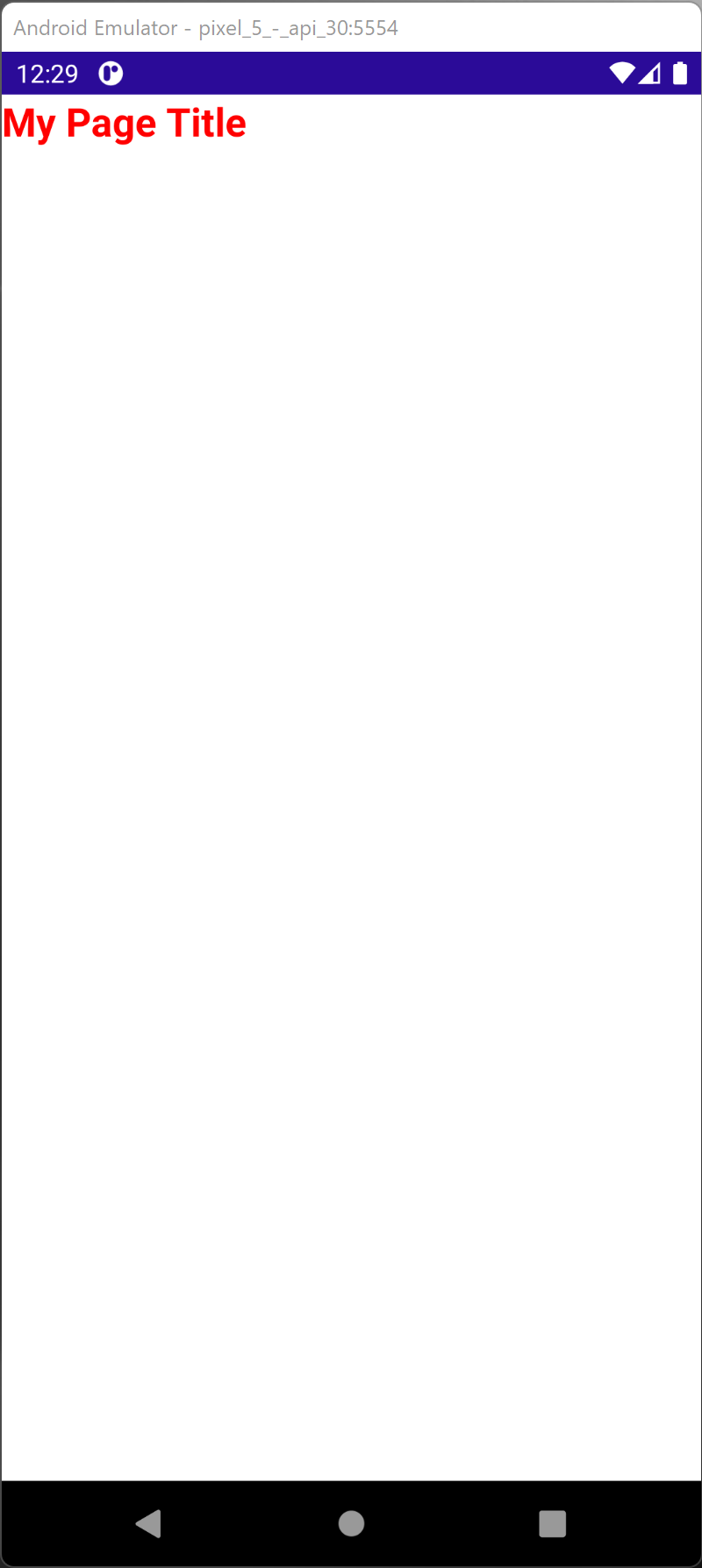
Download